Testing React Native Apps: Strategies, Tools, and Best Practices
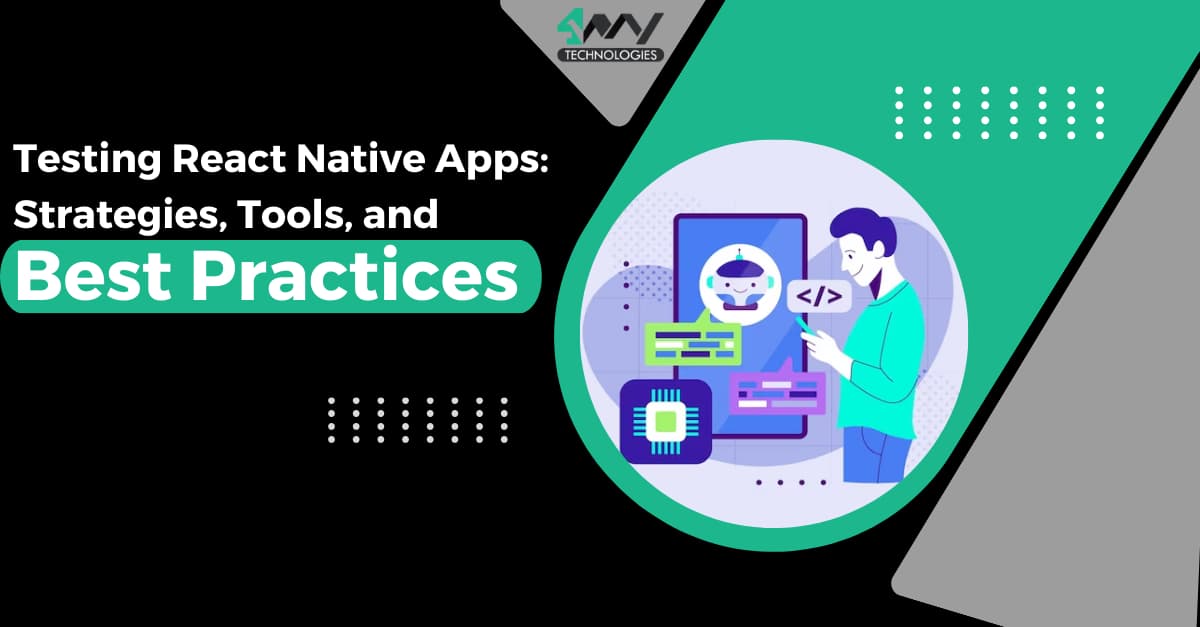
Let us take a deep dive into the dynamic world of React Native app development, where your application's success is dependent on a solid testing strategy. The demand for seamless and dependable experiences has increased as businesses increasingly recognize the critical role of mobile applications. When you hire React Native developers, comprehensive testing skills become essential, and it is appropriate to delve into the core elements that ensure your applications' resilience and performance: testing strategies, tools, and best practices.
This blog post will dissect the complexities of unit testing, integration testing, and end-to-end testing, providing insights into their importance for a React Native developer. We will look at tools like Jest, Detox, React Testing Library, and Enzyme to see how they can help hire React Native developers to build resilient and high-quality applications.
Furthermore, we will go over best practices, such as mocking and stubbing, integrating testing into CI/CD pipelines, and the significance of code coverage metrics. Join me on this journey as we provide you with the knowledge you need to improve your hire React Native developers' testing expertise, ensuring your applications stand the test of time.
Unit Testing: Learning the ABCs of React Native Components
Explanation of Unit Testing in the School of React Native:
Consider React Native to be its own classroom, with one for math, another for language, and so on. Unit testing is now equivalent to giving each classroom (component) a quick quiz to ensure they understand their lessons correctly.
1
2
3
4
// MathComponent.js
export function addNumbers(a, b) {
return a + b;
}
Unit Test for MathComponent:
1
2
3
4
5
6
// MathComponent.test.js
import { addNumbers } from './MathComponent';
test('adds two numbers correctly', () => {
expect(addNumbers(2, 3)).toBe(5); // The quiz: Does the math classroom understand addition?
});
Benefits of Unit Testing: Preventing Silly Mistakes and Ensuring Understanding
1. Preventing Silly Mistakes:
Unit testing, like clearing a small math error before the final exam, helps us catch small mistakes in our code before they become major issues.
2. Ensuring Understanding:
Unit testing ensures that each component of our app, like each student studying a specific subject, functions independently.
Examples of Popular Unit Testing Libraries/Frameworks for React Native
1. Jest: The Quizmaster
Jest acts as a friendly quizmaster, giving quick quizzes to each classroom (component) to check their comprehension. Because of its simplicity and effectiveness, it is widely used in the React Native school.
Installing Jest:
1
npm install --save-dev jest
2. React Testing Library: The Homework Checker
React Testing Library functions similarly to a teacher who checks to see if students (components) understand their homework. It focuses on testing user interactions and behaviors.
Installing React Testing Library:
1
npm install --save-dev @testing-library/react-native
Integration Testing: The School's Teamwork Challenge
Defining Integration Testing in the School of React Native
Integration testing is similar to determining whether different classrooms (components) in our React Native school work well together. It ensures that the math, language, and other classrooms can collaborate without causing chaos during the big school event.
1
2
3
4
5
6
7
8
9
// MathComponent.js
export function addNumbers(a, b) {
return a + b;
}
// LanguageComponent.js
export function greetStudent(name) {
return `Hello, ${name}!`;
}
Integration Test:
1
2
3
4
5
6
7
8
// SchoolEvent.test.js
import { addNumbers } from './MathComponent';
import { greetStudent } from './LanguageComponent';
test('math and language classrooms collaborate smoothly', () => {
const result = greetStudent('Alice') + ' ' + addNumbers(2, 3);
expect(result).toBe('Hello, Alice! 5'); // The teamwork challenge: Do the classrooms collaborate effectively?
});
Significance of Integration Testing: Smooth School Operation
Integration testing ensures that all classrooms (components) can work together without causing chaos, similar to how different classrooms must collaborate for school events in our school. It is critical to ensure that our school runs smoothly and without interruptions.
Discussing the Integration of Various Components/Modules
Real-world Scenario: The School Play
Consider organizing a school play (a difficult feature in our app). Integration testing ensures that each student (component) understands their role and that the play (app feature) runs smoothly without anyone missing a beat. It ensures that the actors (components) work together smoothly to create a successful performance.
When students (components) must collaborate on projects, integration testing ensures that group assignments run smoothly. Just like in real life, it assists in identifying issues prior to the big presentation, ensuring that everyone contributes to the project's success.
End-to-End Testing: The Grand School Performance
Introduction to End-to-End Testing in the School of React Native
End-to-end testing in our React Native school is analogous to organizing a grand school performance in which every student (component) plays a role. It ensures that not only individual students (components) understand their lessons, but that the entire school (app) runs smoothly when presented to the audience (users).
1
2
3
4
5
6
// SchoolPerformance.js
export function organizeGrandPerformance() {
// Code for organizing the grand performance
// Involves coordinating different students (components) for a seamless show
return 'Grand performance successfully organized!';
}
End-to-End Test:
1
2
3
4
5
6
7
// GrandPerformance.test.js
import { organizeGrandPerformance } from './SchoolPerformance';
test('organizing a flawless grand performance', () => {
const result = organizeGrandPerformance();
expect(result).toBe('Grand performance successfully organized!'); // The grand performance: Does the entire school (app) perform flawlessly?
});
Coverage of UI Testing and Interactions: Checking the Stage for a Standing Ovation
End-to-end testing ensures that the stage (UI) looks great and that each actor (user interaction) delivers during the big show. It is like ensuring that all of the decorations, lighting, and actors work together to put on a spectacular show.
1
2
3
4
5
6
7
8
9
// UIComponent.js
export function setStageDecorations(decorations) {
// Code for setting up stage decorations
}
// UserInteraction.js
export function getUserInteractionMarks() {
// Code for tracking user interaction marks
}
End-to-End Test for UI and User Interactions:
1
2
3
4
5
6
7
8
// GrandPerformance.test.js
import { setStageDecorations, getUserInteractionMarks } from './UIComponent';
test('checking the stage and user interactions for a standing ovation', () => {
setStageDecorations('Amazing Decorations');
const userInteractionMarks = getUserInteractionMarks();
expect(userInteractionMarks).toBe('Excellent'); // Checking if the stage looks great and user interactions are flawless
});
Tools and Frameworks Suitable for Implementing End-to-End Testing: Detox
Detox is a tool for end-to-end testing in React Native, much like a director ensures every actor follows the script and performs well during the grand performance. It oversees the entire performance, ensuring that every component works in unison.
Detox Installation:
1
npm install --save-dev detox
Jest: The Friendly Quizmaster
Overview of Jest in the School of React Native
Jest acts as the friendly quizmaster in our React Native school, ensuring that every classroom (component) understands its lessons. It is a well-known testing framework that streamlines the testing process and ensures our students (components) are well-prepared for the big exam (app launch).
Key Features: Snapshot Testing and Parallel Test Execution
Snapshot Testing:
1
2
3
4
5
6
7
// MathComponent.test.js
import { addNumbers } from './MathComponent';
test('snapshot test for adding numbers', () => {
const result = addNumbers(2, 3);
expect(result).toMatchSnapshot(); // Jest takes a snapshot of the result
});
Parallel Test Execution:
1
2
3
4
5
6
// LanguageComponent.test.js
import { greetStudent } from './LanguageComponent';
test('greeting a student in multiple languages', () => {
expect(greetStudent('Alice')).toBe('Hello, Alice!'); // Multiple tests running in parallel
});
Integration with React Native Projects:
1
npm install --save-dev jest
Detox: The Director for Grand Performances
Understanding Detox in the School of React Native:
Detox is like the director of our React Native school's grand performance. It specializes in end-to-end testing, ensuring that each actor (component) follows the script and performs well during the big show (app feature).
Features: Automatic Synchronization and Rich API
Automatic Synchronization
1
2
3
4
5
6
7
// GrandPerformance.test.js
import { organizeGrandPerformance } from './SchoolPerformance';
test('organizing a flawless grand performance with Detox', async () => {
await device.reloadReactNative(); // Automatic synchronization with the app
await expect(organizeGrandPerformance()).toBe('Grand performance successfully organized!');
});
Rich API
1
2
3
4
5
6
7
8
9
// UserInteraction.js
export function simulateUserInteraction() {
// Code for simulating user interaction
}
// GrandPerformance.test.js
test('simulating user interaction during the grand performance', async () => {
await simulateUserInteraction(); // Detox's rich API for simulating user interactions
});
Setting Up Detox in a React Native Project:
1
npm install --save-dev detox
Writing Test Cases with Detox:
1
npx detox init
React Testing Library: The Homework Checker
Role of React Testing Library in the School of React Native
React Testing Library functions similarly to a teacher checking to see if students (components) understand their homework. It focuses on unit and integration testing, with a particular emphasis on user interactions and behaviors.
Emphasis on Testing User Interactions
1
2
3
4
5
6
7
8
9
// UserInteraction.test.js
import { render, fireEvent } from '@testing-library/react-native';
import { ButtonComponent } from './ButtonComponent';
test('clicking a button triggers the expected behavior', () => {
const { getByText } = render(<ButtonComponent />);
fireEvent.press(getByText('Click me')); // Testing user interaction
// Assertion for the expected behavior
});
Enzyme: The Testing Utility for React Components
Introducing Enzyme in the School of React Native
Enzyme is a special tool in our React Native school that allows us to inspect and interact with our React components. It offers various rendering approaches, ensuring that our components are thoroughly tested.
Shallow Rendering and Full Rendering
1
2
3
4
5
6
7
8
9
10
11
12
13
// ButtonComponent.test.js
import { shallow, mount } from 'enzyme';
import { ButtonComponent } from './ButtonComponent';
test('shallow rendering for a button component', () => {
const wrapper = shallow(<ButtonComponent />);
expect(wrapper.exists()).toBe(true); // Shallow rendering checks if the component renders without deeply rendering child components
});
test('full rendering for a button component', () => {
const wrapper = mount(<ButtonComponent />);
expect(wrapper.find('button').text()).toBe('Click me'); // Full rendering interacts with child components and checks their behavior
});
Tips for Effective Testing Using Enzyme
- Use Shallow Rendering Wisely: Shallow rendering is useful for testing isolated components.
- Use Full Rendering for Interactions: When testing interactions with child components, use full rendering.
Mocking and Stubbing: Setting the Stage for a Flawless School Play
The Importance of Mocking and Stubbing in Testing
Consider organizing a school play (app feature) with various actors (components) and props (external services). Mocking and stubbing are similar to rehearsing stand-in actors and props to ensure everything runs smoothly during the actual performance. These techniques are used in React Native testing to simulate external services or components in order to focus on specific scenarios without involving the entire production.
Effective Mocks and Stubs in React Native Tests
1. Mocking External Services
1
2
3
4
5
6
7
8
9
10
11
12
// ApiService.js
export function fetchData() {
// Code to fetch data from an external API
}
// ComponentUsingApi.js
import { fetchData } from './ApiService';
export function useData() {
const data = fetchData();
// Code that uses the fetched data
}
Mocking External Service in the Test:
1
2
3
4
5
6
7
8
9
// ComponentUsingApi.test.js
import { fetchData } from './ApiService';
jest.mock('./ApiService');
test('testing component behavior with mocked API data', () => {
fetchData.mockReturnValue('Mocked API Data');
const result = useData();
expect(result).toBe('Mocked API Data'); // Ensuring the component behaves as expected with mocked data
});
2. Stubbing Function Calls:
1
2
3
4
5
6
7
8
9
10
11
12
// MathService.js
export function addNumbers(a, b) {
// Code to add two numbers
}
// ComponentUsingMath.js
import { addNumbers } from './MathService';
export function useSum() {
const sum = addNumbers(2, 3);
// Code that uses the calculated sum
}
Stubbing Function Call in the Test:
1
2
3
4
5
6
7
8
// ComponentUsingMath.test.js
import { addNumbers } from './MathService';
jest.spyOn(MathService, 'addNumbers').mockReturnValue(5);
test('testing component behavior with stubbed math function', () => {
const result = useSum();
expect(result).toBe(5); // Ensuring the component behaves as expected with the stubbed math function
});
Real-world Examples Demonstrating the Use of Mocks and Stubs
Scenario 1: Testing a Login Component with Authentication
1
2
3
4
5
6
7
8
9
10
11
12
// AuthService.js
export function authenticateUser(username, password) {
// Code to authenticate user credentials
}
// LoginComponent.js
import { authenticateUser } from './AuthService';
export function loginUser(username, password) {
const isAuthenticated = authenticateUser(username, password);
// Code that handles login based on authentication status
}
Mocking Authentication in the Test:
1
2
3
4
5
6
7
8
9
// LoginComponent.test.js
import { authenticateUser } from './AuthService';
jest.mock('./AuthService');
test('testing login component behavior with mocked authentication', () => {
authenticateUser.mockReturnValue(true);
const result = loginUser('user123', 'pass456');
expect(result).toBe('Login Successful'); // Ensuring the login component behaves as expected with mocked authentication
});
Scenario 2: Testing a Weather App Component with External API
1
2
3
4
5
6
7
8
9
10
11
12
// WeatherService.js
export function fetchWeatherData(city) {
// Code to fetch weather data from an external API
}
// WeatherComponent.js
import { fetchWeatherData } from './WeatherService';
export function displayWeather(city) {
const weatherData = fetchWeatherData(city);
// Code that displays weather information
}
Mocking Weather Data in the Test:
1
2
3
4
5
6
7
8
9
// WeatherComponent.test.js
import { fetchWeatherData } from './WeatherService';
jest.mock('./WeatherService');
test('testing weather component behavior with mocked weather data', () => {
fetchWeatherData.mockReturnValue({ temperature: 25, condition: 'Sunny' });
const result = displayWeather('New York');
expect(result).toBe('Temperature: 25°C, Condition: Sunny'); // Ensuring the weather component behaves as expected with mocked weather data
});
Continuous Integration/Continuous Deployment (CI/CD): The Assembly Line
Integration of Testing into CI/CD Pipelines for React Native Apps
In React Native, CI/CD functions as an efficient assembly line for developing and delivering apps. Instead of assembling each project by hand, CI/CD automates the process, ensuring that every classroom (component) works in unison.
Example CI/CD Pipeline Configuration:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
# .gitlab-ci.yml
stages:
- test
- build
- deploy
test:
stage: test
script:
- npm install
- npm test
build:
stage: build
script:
- npm install
- npm run build
deploy:
stage: deploy
script:
- npm install
- npm run deploy
The pipeline in this configuration has three stages: testing, building, and deploying. Before proceeding to the next stages, we must ensure that our classrooms (components) pass their tests.
Benefits of Automated Testing in CI/CD Workflows
1. Early Detection of Issues
In CI/CD, automated testing ensures that any issues or mistakes in our classrooms (components) are identified early in the assembly line. Identifying issues early saves time and ensures quality, just like identifying a typo in homework before submitting it.
2. Consistent Performance:
CI/CD ensures that each project (app version) adheres to the same quality standards. It is like having a standardized process for all school projects, ensuring consistent performance.
3. Faster Delivery:
With automated testing, projects (apps) move more smoothly through the assembly line, reducing manual effort. This rate of delivery is comparable to efficiently completing and presenting multiple school projects in a short period of time.
Examples of Popular CI/CD Tools Compatible with React Native
1. GitLab CI/CD: The School Project Manager
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
# .gitlab-ci.yml
stages:
- test
- build
- deploy
test:
stage: test
script:
- npm install
- npm test
build:
stage: build
script:
- npm install
- npm run build
deploy:
stage: deploy
script:
- npm install
- npm run deploy
2. GitHub Actions: The Homework Checker Assistant
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
# .github/workflows/main.yml
name: CI/CD Workflow
on:
push:
branches:
- main
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout Repository
uses: actions/checkout@v2
- name: Install Dependencies and Run Tests
run: |
npm install
npm test
3. Jenkins: The All-in-One School Organizer
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
// Jenkinsfile
pipeline {
agent any
stages {
stage('Test') {
steps {
script {
sh 'npm install'
sh 'npm test'
}
}
}
stage('Build') {
steps {
script {
sh 'npm install'
sh 'npm run build'
}
}
}
stage('Deploy') {
steps {
script {
sh 'npm install'
sh 'npm run deploy'
}
}
}
}
}
Code Coverage: Ensuring Every Student's Lesson is Checked
Importance of Code Coverage Metrics in Testing React Native Apps
Code coverage in our React Native school is equivalent to determining how much of each student's (component's) lesson has been reviewed. It ensures that every aspect of the school's curriculum (codebase) is tested, reducing the possibility of missing potential issues.
1
2
3
4
5
6
7
8
9
10
11
// MathComponent.js
export function addNumbers(a, b) {
return a + b;
}
// MathComponent.test.js
import { addNumbers } from './MathComponent';
test('adds two numbers correctly', () => {
expect(addNumbers(2, 3)).toBe(5);
});
Tools for Measuring Code Coverage and Interpreting Results
1. Jest Coverage: The Lesson Checker
1
2
3
4
5
6
7
npm install --save-dev jest
// package.json
{
"scripts": {
"test": "jest --coverage"
}
}
When you run npm test, Jest will provide a coverage report, showing how much of your code is tested.
2. Istanbul/NYC: The School Inspector
1
2
3
4
5
6
7
npm install --save-dev nyc
// package.json
{
"scripts": {
"test": "nyc --reporter=text-summary jest"
}
}
NYC collaborates with Jest to generate detailed coverage reports, allowing you to see which parts of your code are well-covered and which require additional attention.
Strategies for Improving Code Coverage in a React Native Project
1. Set Coverage Goals: The Learning Targets
1
2
3
4
5
6
7
8
9
10
11
12
13
14
# Set a coverage threshold in Jest
# package.json
{
"jest": {
"coverageThreshold": {
"global": {
"branches": 90,
"functions": 95,
"lines": 95,
"statements": 95
}
}
}
}
Set specific coverage percentage targets. This, like setting goals for each student's grade, ensures a thorough review of your code.
2. Identify Uncovered Areas: The Homework Feedback
1
2
3
4
5
6
7
# Istanbul/NYC with Jest
# package.json
{
"scripts": {
"test": "nyc --reporter=text-summary --reporter=html jest"
}
}
Create HTML reports to visually identify areas that require additional attention, similar to reviewing homework and providing detailed feedback.
Conclusion
In this blog, we experimented with a variety of strategies and tools, such as Jest for testing lessons, Detox for grand performances, and CI/CD pipelines for efficient project assembly. Code coverage serves as the diligent school inspector, ensuring that all components are thoroughly checked.
Developer React Native must remain curious as the React Native testing landscape evolves, exploring new tools and adapting to emerging best practices. React Native Developers, like students, can improve their skills and contribute to the growing success of React Native in the world of mobile app development. Happy coding!

A science graduate who has a keen interest to lean about new technologies and research area. With an experience in the field of data analytics and content writing, she aims to share her knowledge among passionate tech readers.